Ever since React established itself as an essential tool in the modern Front-end, rendering strategies have always been a point of attention in the development community.
Traditionally, a React Single-Page Application (SPA) starts by sending an empty element <div> to the browser, along with a larger JavaScript package. This package includes the React and application code, while the client is responsible for: fetching the data, processing the interface, and making it interactive. This process is known as Client-Side Rendering (CRS).
Although functional, this approach can result in slow loading times and lower than expected performance, especially on more limited devices.
Fortunately, React has involved and incorporated significant contributions from the community to improve rendering performance. One of these innovations was React Server Components (RSCs), which offer a way of rendering parts of the interface on the server, reducing the work on the client.
What are React Server Components?
React Server Components allow you to create components that run and render on the server, while others components remain on the client to handle interactivity. They introduce a new layer of efficiency by combining the benefits of server and client rendering.
With Server Components, logic such as data fetching or formatting is processed on the server. This eliminates the need for unnecessary round-trips between client and server, delivering pre-rendered HTML and optimizing performance.
Basic example of a Server Component:
import fetchDataFromAPI from './api';
export default async function ServerComponent() {
const data = await fetchDataFromAPI();
return (
<div>
<h1>Data from the server:</h1>
<ul>
{data.map((item) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
}
This component fetches the data from the server and returns a ready-made HTML, reducing the load on the client.
Advantages of React Server Components
Best performance
Reduces the size of the JavaScript sent to the client, reducing the initial loading time.
Eliminates the need for complete “hydration” of rendered HTML on the server.
Optimized SEO
As the HTML is generated on the server, the search engines are able to index the pages efficiently.
Increased security
Sensitive information, such as authentication tokens, remains on the server and is not exposed to the client.
Simplified development
Centralized data search and transformation logic on the server reduces complexity on the client.
Differences between Server Components and Client Components
Server Components:
They run on the server.
They don’t have access to the browser’s DOM or APIs. (e.g. window or document)
Perfect for operations such as searching for data and generating static content.
Client Components:
They run on the client.
Handle interactivity, such as client events and forms.
They can consume Server Components to display pre-rendered content.
Integration example:
// ServerComponent.tsx
import ClientComponent from "./ClientComponent";
interface Item { id: number; title: string; }
export default async function ServerComponent() {
const data: Item[] = await fetch('https://api.example.com/data').then((res) => res.json());
return (
<div>
<ClientComponent data={data} />
</div>
);
}
// ClientComponent.tsx
import { useState, useEffect } from 'react';
import ServerComponent from './ServerComponent';
interface Item { id: number; title: string; }
interface ClientComponentProps { data: Item[]; }
export default function ClientComponent({ data}: ClientComponentProps) {
const [items, setItems] = useState<Item[]>([]);
useEffect(() => {
setItems(data)
}, [data]);
return (
<ul>
{items.map((item) => (
<li key={item.id}>{item.title}</li>
))}
</ul>
);
}
In the example above, the client component displays the list of items as soon as the data is received from the server and stored in the local state.
Good Practices
Clear divisions of responsibilities
Use Server Components for non-interactive tasks and Client Components for DOM manipulation.
Avoid unnecessary re-rendering
Plan the component hierarchy to minimize customer work
Test and monitoring
Use tools like React DevTools to identify bottlenecks and optimize performance.
Security
Always validate and sanitize your data on the server to avoid vulnerabilities.
Conclusion
React Server Components represent a significant evolution in the way we build web applications. They allow us to combine the best of both worlds: efficiency and simplicity on the server with interactivity and dynamism on the client.
Adopting this approach can not only improve the performance of your project, but also simplify its architecture. Try incorporating Server Components into your next project and enjoy the benefits of a faster, more efficient web.
Tags
Subscribe to
Our
Newsletter
Join 1,000+ people and recieve our weekly insights.
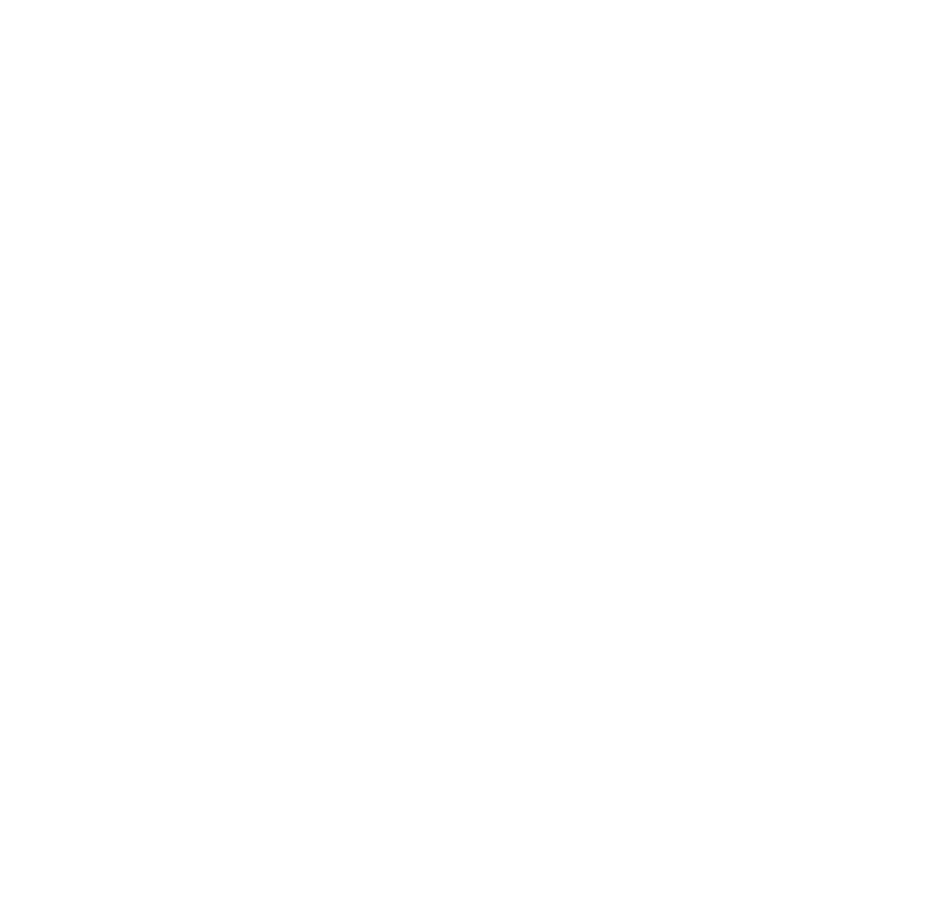
Success!
Thank your for subscribing to Buzzvel's
Newsletter, you will now
receive
amazing
tips
and insights weekly.